First some basic rules.
- Some tokens require a matching token and some do not.
- A matching start token must be matched with an end token.
- Matching tokens must be nested. If not, an error occurred.
Matching Tokens Example |
1 |
Start parsing a string. The LIFO stack is empty |
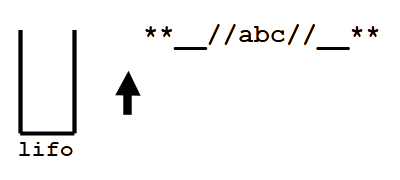 |
5 |
A token that needs to be matched
is found. If it matches the top of the stack,
pop the token off the stack. |
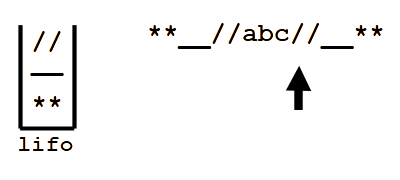 |
2 |
A token that needs to be matched
is found. If it does not match the top of stack,
push the token onto the stack. |
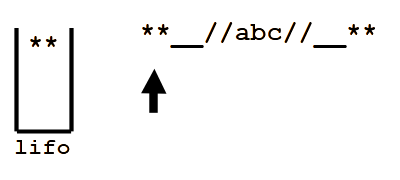 |
6
A token that needs to be matched
is found. If it matches the top of the stack,
pop the token off the stack. |
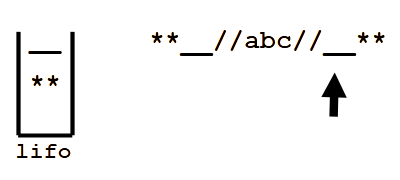 |
3 |
A token that needs to be matched
is found. If it does not match the top of stack,
push the token onto the stack. |
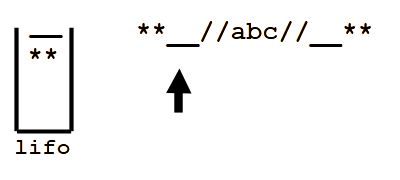 |
7 |
A token that needs to be matched
is found. If it matches the top of the stack,
pop the token off the stack. |
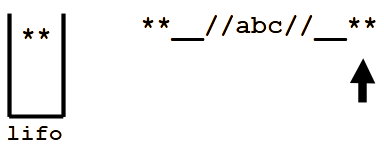 |
4 |
A token that needs to be matched
is found. If it does not match the top of stack,
push the token onto the stack. |
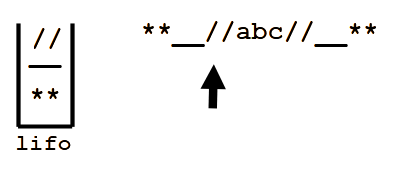 |
8 |
End parsing the string. The LIFO stack is empty. Success! |
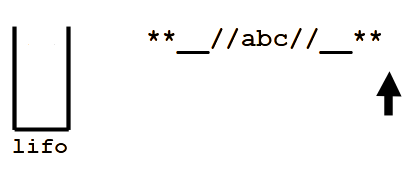 |
|
Note: If there is a token left on the top of the
stack an error has occurred.
There was no matching token found.
(The exception may be a paragraph token.)
#!/usr/bin/python3
# =============================================================
# From: www.youtube.com/watch?v=34SNQHapJYE
# A Very Fast And Memory Efficient Alternative To
# Python Lists (Deque)
#
# Queue.queue vs Collections.deque ----------------------------
# Queue.queue is used to communicate between threads.
# Collections.deque is used as a data structure within
# a thread to perform certain functionality.
#
# Types of queues ---------------------------------------------
# LIFO - Last End First Out (often called a stack)
# FIFO - First In First Out
# =============================================================
from collections import deque
print()
print(' <-- front of Q end of Q -->')
print()
people = ['Mario','Luigi','Toad']
queue = deque(people)
queue.append('Bowser') # add to Q end (end of Q)
print(f'[1] {queue}')
queue.appendleft('Dasiy') # add to Q beginning (front of Q)
print(f'[2] {queue}')
queue.popleft() # pop from front of Q
print(f'[3] {queue}')
queue.pop() # pop from end of Q
print(f'[4] {queue}')
queue.rotate(-1) # rotate Q left
print(f'[5] {queue}')
queue.rotate(2) # rotate Q right
print(f'[6] {queue}')
queue.extend(['Tom','Dick','Harry'])
print(f'[7] {queue}')
queue.extendleft(['Judy','Jane','Mary'])
print(f'[8] {queue}')
queue.reverse() # rotate Q
print(f'[9] {queue}')
#!/usr/bin/python3
# =============================================================
# my LIFO queue/stack using a list
# (why do it myself - because I wanted to)
# =============================================================
from copy import deepcopy
# -------------------------------------------------------------
# ---- my LIFO queue/stack
# -------------------------------------------------------------
class MyLifoQueue():
def __init__(self):
self.lifo = []
def empty(self):
l = len(self.lifo)
if l < 1:
return True
return False
def length(self):
return(len(self.lifo))
def pop(self):
if len(self.lifo) > 0:
obj = self.lifo.pop(-1)
return obj
else:
return None
def push(self,obj):
self.lifo.append(obj)
def copy(self):
if len(self.lifo) < 1:
return None
return deepcopy(self.lifo[-1])
def state(self):
if len(self.lifo) < 1:
return -1
return self.lifo[-1][0]
def dump(self):
l = len(self.lifo)
print(f'lifo length {l}')
if l < 1:
return
top = True # mark top of queue?
i = 0
while l:
l -= 1
obj = self.lifo[l]
if top:
print(f'[{i:2}] {obj} (top of queue)')
top = False
else:
print(f'[{i:2}] {obj}')
i += 1